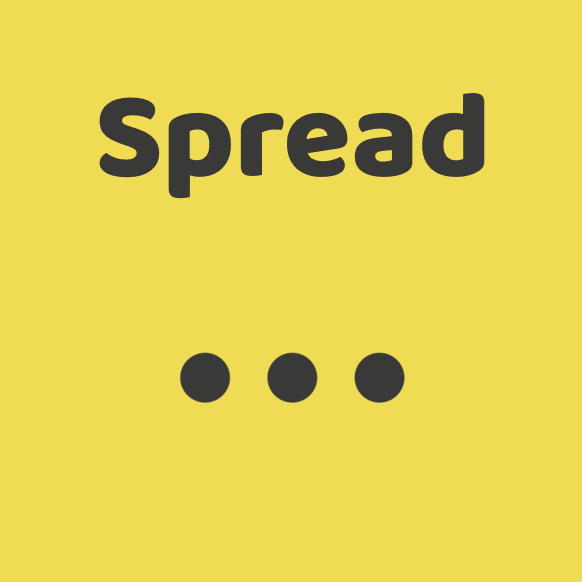
์คํ๋ ๋ ์ฐ์ฐ์๋?
์คํ๋ ๋ ์ฐ์ฐ์(Spread Operator)๋ ES6์์ ๋์
๋ ๊ธฐ๋ฅ์ผ๋ก, ๋ฐฐ์ด์ด๋ ๊ฐ์ฒด๋ฅผ ํผ์น๋ ์ญํ ์ ํฉ๋๋ค. ์ด ์ฐ์ฐ์๋ ์ธ ๊ฐ์ ์ ( ...
)์ผ๋ก ํํ๋๋ฉฐ, ๋ค์ํ ์ํฉ์์ ์ ์ฉํ๊ฒ ์ฌ์ฉ๋ ์ ์์ต๋๋ค.
์ฃผ์ ์ฉ๋
1. ๋ฐฐ์ด ๋ณต์ฌ ๋ฐ ๋ณํฉ
const arr1 = [1, 2, 3];
const arr2 = [...arr1, 4, 5]; // [1, 2, 3, 4, 5]
const combined = [...arr1, ...arr2]; // [1, 2, 3, 1, 2, 3, 4, 5]
2. ๊ฐ์ฒด ๋ณต์ฌ ๋ฐ ๋ณํฉ
const obj1 = { foo: 'bar', x: 42 };
const obj2 = { foo: 'baz', y: 13 };
const clonedObj = { ...obj1 }; // { foo: 'bar', x: 42 }
const mergedObj = { ...obj1, ...obj2 }; // { foo: 'baz', x: 42, y: 13 }
3. ํจ์ ์ธ์๋ก ์ ๋ฌ
function sum(x, y, z) {
return x + y + z;
}
const numbers = [1, 2, 3];
console.log(sum(...numbers)); // 6
์ฃผ์์ฌํญ
1. ์คํ๋ ๋ ์ฐ์ฐ์๋ ์์ ๋ณต์ฌ(Shallow Copy)๋ง ์ํํฉ๋๋ค. ๊ฐ์ฒด๋ ๋ฐฐ์ด์ ์ต์์ ์์ค์ ์์ฑ๋ง ๋ณต์ฌ๋๋ฉฐ, ์ค์ฒฉ๋ ๋ฐฐ์ด์ด๋ ๊ฐ์ฒด์ ๋ํด์๋ ์ฐธ์กฐ๋ง ๋ณต์ฌ๋ฉ๋๋ค. ๋ฐ๋ผ์ ๋ณต์ฌ๋ ๊ฐ์ฒด๋ ๋ฐฐ์ด ๋ด์ ์ค์ฒฉ๋ ๊ฐ์ฒด๋ ๋ฐฐ์ด์ ์๋ณธ๊ณผ ๋์ผํ ๊ฐ์ฒด๋ฅผ ๊ฐ๋ฆฌํต๋๋ค.
// ๋ฐฐ์ด์ ๊ฒฝ์ฐ
const originalArray = [1, [2, 3]];
const copiedArray = [...originalArray];
copiedArray[0] = 10; // ์ต์์ ์์๋ ๋
๋ฆฝ์
copiedArray[1][0] = 20; // ์ค์ฒฉ๋ ๋ฐฐ์ด์ ์ฐธ์กฐ๊ฐ ๋ณต์ฌ๋จ
console.log(originalArray); // [1, [20, 3]]
console.log(copiedArray); // [10, [20, 3]]
2. ๊ฐ์ฒด์ ์ฌ์ฉํ ๋, ์ค๋ณต๋ ํค๊ฐ ์๋ค๋ฉด ๋์ค์ ์ค๋ ๊ฐ์ด ์ด์ ๊ฐ์ ๋ฎ์ด์๋๋ค.
ํ์ฉ ์์
1. ๋ฐฐ์ด ์์ ์ถ๊ฐ/์ ๊ฑฐ
const fruits = ['apple', 'banana'];
const moreFruits = ['mango', ...fruits, 'orange']; // ['mango', 'apple', 'banana', 'orange']
const [first, ...rest] = moreFruits;
console.log(first); // 'mango'
console.log(rest); // ['apple', 'banana', 'orange']
2. ๋ฌธ์์ด์ ๊ฐ๋ณ ๋ฌธ์๋ก ๋ถ๋ฆฌ
const str = "Hello";
const chars = [...str]; // ['H', 'e', 'l', 'l', 'o']
3. ๋ฐฐ์ด ์ค๋ณต ์ ๊ฑฐํ๊ธฐ
const arr = [1, 2, 3, 2, 4, 4]
const uniqueArr = [...new Set(arr)]; // [1, 2, 3, 4]
4. ๋ฐฐ์ด ๋ด ์ต๋/์ต์๊ฐ ์ฐพ๊ธฐ
const numbers = [5, 2, 8, 1, 9];
const max = Math.max(...numbers); // 9
const min = Math.min(...numbers); // 1
5. ์กฐ๊ฑด๋ถ๋ก ๊ฐ์ฒด ์์ฑ ์ถ๊ฐ
const addUser = (age) => {
return {
name: "Yundogi",
...(age > 18 && { canVote: true })
};
};
console.log(addUser(26)) // {name: 'Yundogi', canVote: true}
6. NodeList ๋ฐฐ์ด๋ก ๋ฐํ
const divs = document.querySelectorAll('div');
const divsArray = [...divs];
'๐ฅ Develop > Javascript' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[Javascript] ๋ฐฐ์ด๊ณผ ๊ฐ์ฒด ์ ๋ ฌํ๊ธฐ (0) | 2024.07.29 |
---|---|
[Javascript] Object.entries() ๋ฉ์๋ (0) | 2024.07.27 |
[Javascript] ๋ฐ์ดํฐ ํ์ ์ ๋ฉ๋ชจ๋ฆฌ ์ฐธ์กฐ ๋ฐฉ์ (1) | 2024.07.24 |
[Javascript] ํด๋ก์ (Closure) (3) | 2024.07.20 |
[Javascript] ํธ์ด์คํ (Hoisting) (0) | 2024.07.19 |
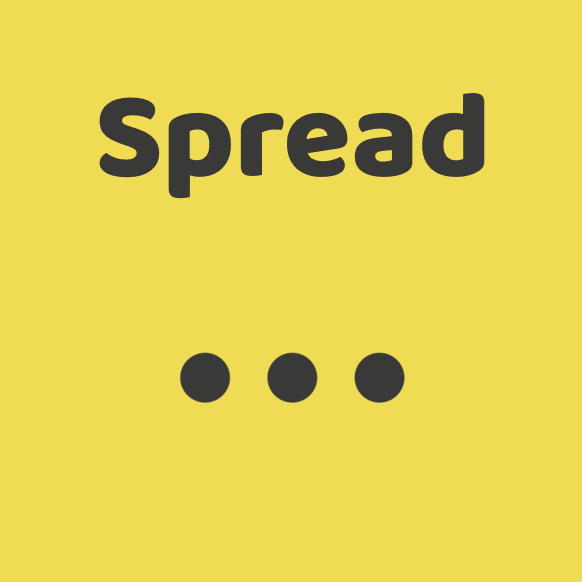
์คํ๋ ๋ ์ฐ์ฐ์๋?
์คํ๋ ๋ ์ฐ์ฐ์(Spread Operator)๋ ES6์์ ๋์
๋ ๊ธฐ๋ฅ์ผ๋ก, ๋ฐฐ์ด์ด๋ ๊ฐ์ฒด๋ฅผ ํผ์น๋ ์ญํ ์ ํฉ๋๋ค. ์ด ์ฐ์ฐ์๋ ์ธ ๊ฐ์ ์ ( ...
)์ผ๋ก ํํ๋๋ฉฐ, ๋ค์ํ ์ํฉ์์ ์ ์ฉํ๊ฒ ์ฌ์ฉ๋ ์ ์์ต๋๋ค.
์ฃผ์ ์ฉ๋
1. ๋ฐฐ์ด ๋ณต์ฌ ๋ฐ ๋ณํฉ
const arr1 = [1, 2, 3];
const arr2 = [...arr1, 4, 5]; // [1, 2, 3, 4, 5]
const combined = [...arr1, ...arr2]; // [1, 2, 3, 1, 2, 3, 4, 5]
2. ๊ฐ์ฒด ๋ณต์ฌ ๋ฐ ๋ณํฉ
const obj1 = { foo: 'bar', x: 42 };
const obj2 = { foo: 'baz', y: 13 };
const clonedObj = { ...obj1 }; // { foo: 'bar', x: 42 }
const mergedObj = { ...obj1, ...obj2 }; // { foo: 'baz', x: 42, y: 13 }
3. ํจ์ ์ธ์๋ก ์ ๋ฌ
function sum(x, y, z) {
return x + y + z;
}
const numbers = [1, 2, 3];
console.log(sum(...numbers)); // 6
์ฃผ์์ฌํญ
1. ์คํ๋ ๋ ์ฐ์ฐ์๋ ์์ ๋ณต์ฌ(Shallow Copy)๋ง ์ํํฉ๋๋ค. ๊ฐ์ฒด๋ ๋ฐฐ์ด์ ์ต์์ ์์ค์ ์์ฑ๋ง ๋ณต์ฌ๋๋ฉฐ, ์ค์ฒฉ๋ ๋ฐฐ์ด์ด๋ ๊ฐ์ฒด์ ๋ํด์๋ ์ฐธ์กฐ๋ง ๋ณต์ฌ๋ฉ๋๋ค. ๋ฐ๋ผ์ ๋ณต์ฌ๋ ๊ฐ์ฒด๋ ๋ฐฐ์ด ๋ด์ ์ค์ฒฉ๋ ๊ฐ์ฒด๋ ๋ฐฐ์ด์ ์๋ณธ๊ณผ ๋์ผํ ๊ฐ์ฒด๋ฅผ ๊ฐ๋ฆฌํต๋๋ค.
// ๋ฐฐ์ด์ ๊ฒฝ์ฐ
const originalArray = [1, [2, 3]];
const copiedArray = [...originalArray];
copiedArray[0] = 10; // ์ต์์ ์์๋ ๋
๋ฆฝ์
copiedArray[1][0] = 20; // ์ค์ฒฉ๋ ๋ฐฐ์ด์ ์ฐธ์กฐ๊ฐ ๋ณต์ฌ๋จ
console.log(originalArray); // [1, [20, 3]]
console.log(copiedArray); // [10, [20, 3]]
2. ๊ฐ์ฒด์ ์ฌ์ฉํ ๋, ์ค๋ณต๋ ํค๊ฐ ์๋ค๋ฉด ๋์ค์ ์ค๋ ๊ฐ์ด ์ด์ ๊ฐ์ ๋ฎ์ด์๋๋ค.
ํ์ฉ ์์
1. ๋ฐฐ์ด ์์ ์ถ๊ฐ/์ ๊ฑฐ
const fruits = ['apple', 'banana'];
const moreFruits = ['mango', ...fruits, 'orange']; // ['mango', 'apple', 'banana', 'orange']
const [first, ...rest] = moreFruits;
console.log(first); // 'mango'
console.log(rest); // ['apple', 'banana', 'orange']
2. ๋ฌธ์์ด์ ๊ฐ๋ณ ๋ฌธ์๋ก ๋ถ๋ฆฌ
const str = "Hello";
const chars = [...str]; // ['H', 'e', 'l', 'l', 'o']
3. ๋ฐฐ์ด ์ค๋ณต ์ ๊ฑฐํ๊ธฐ
const arr = [1, 2, 3, 2, 4, 4]
const uniqueArr = [...new Set(arr)]; // [1, 2, 3, 4]
4. ๋ฐฐ์ด ๋ด ์ต๋/์ต์๊ฐ ์ฐพ๊ธฐ
const numbers = [5, 2, 8, 1, 9];
const max = Math.max(...numbers); // 9
const min = Math.min(...numbers); // 1
5. ์กฐ๊ฑด๋ถ๋ก ๊ฐ์ฒด ์์ฑ ์ถ๊ฐ
const addUser = (age) => {
return {
name: "Yundogi",
...(age > 18 && { canVote: true })
};
};
console.log(addUser(26)) // {name: 'Yundogi', canVote: true}
6. NodeList ๋ฐฐ์ด๋ก ๋ฐํ
const divs = document.querySelectorAll('div');
const divsArray = [...divs];
'๐ฅ Develop > Javascript' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[Javascript] ๋ฐฐ์ด๊ณผ ๊ฐ์ฒด ์ ๋ ฌํ๊ธฐ (0) | 2024.07.29 |
---|---|
[Javascript] Object.entries() ๋ฉ์๋ (0) | 2024.07.27 |
[Javascript] ๋ฐ์ดํฐ ํ์ ์ ๋ฉ๋ชจ๋ฆฌ ์ฐธ์กฐ ๋ฐฉ์ (1) | 2024.07.24 |
[Javascript] ํด๋ก์ (Closure) (3) | 2024.07.20 |
[Javascript] ํธ์ด์คํ (Hoisting) (0) | 2024.07.19 |